Master Core Java Interview Questions and Answers (2025) – Ultimate Guide to Success
Welcome to the Ultimate Core Java Interview Questions and Answers Guide!
Whether you’re an experienced Java developer or just starting your career in Java programming, this resource is tailored to help you succeed in your interviews and strengthen your understanding of key Java concepts.
We have compiled a list of frequently asked Java interview questions, designed to cover a wide range of core Java topics. Our goal is to provide comprehensive Java interview questions and answers for developers at all levels, including beginners with experience developers.
Key topics include:
- Java basics
- Object-oriented programming (OOP) concepts in Java
- Core Java interview questions for different experience levels
- Core Java syllabus and essential topics
Table of Contents
Toggle1. What is Java?
Java is a high-level programming language created by James Gosling in 1991. It enables developers to create software that runs across various platforms and devices, including websites and mobile applications.
2. What are the Features of Java?
- Simple: Java is easy to learn, making it accessible for developers of all skill levels.
- Object-Oriented: Everything in Java is treated as an object, with properties and behaviors. Developers can manipulate these through classes and objects.
- Platform-Independent: Write once, run anywhere. Java code runs on all operating systems like Windows, Linux, Unix, and macOS.
- Portable: Java bytecode can run on any system, ensuring code portability across platforms.
- Secure: Java uses internal pointers, which hide object memory locations for enhanced security.
- Robust: Java’s strong memory management and automatic garbage collection make it a stable language.
- Multithreading: Java supports multiple tasks running concurrently with shared memory.
- Architecture-Neutral: Java is independent of system architecture.
- Distributed: Java supports distributed systems like RMI and EJB.
3. What is OOP?
OOP stands for Object-Oriented Programming. It focuses on creating programs using classes and objects. Here are its main concepts:
- Object: An instance that contains data (state) and behavior. Examples include chairs, pens, and bicycles.
- Class: A blueprint for objects that contains variables, methods, and constructors.
- Inheritance: Child classes inherit properties and methods from parent classes.
- Polymorphism: Performing a single task in different ways, such as method overloading and overriding.
- Abstraction: Hiding implementation details while showing only functionality, like using a phone to call without knowing how it works internally.
- Encapsulation: Binding data and code into a single unit, such as a Model class in Java.
4. What is a Constructor?
A constructor is a special method used to initialize objects in Java. It shares the class name, has no return type, and cannot use keywords like abstract
, static
, or final
.
Types of Constructors:
- Default Constructor: Parameter-less constructor that initializes default values.
- Parameterized Constructor: Accepts parameters to initialize objects with specific values.
Constructor Chaining:
This refers to one constructor calling another. It can happen within the same class using the this
keyword or between different classes using the super
keyword.
5. What is an Abstract Class?
An abstract class in Java is a class that contains abstract methods (methods without a body) as well as concrete methods. It provides partial abstraction and is used to define common behavior for subclasses.
Achieving Abstraction:
- Abstract Class: Offers 0-100% abstraction.
- Interface: Offers 100% abstraction.
6. What is Association and Composition?
Association refers to relationships between two or more objects. It comes in two types:
- Aggregation: A weak relationship where objects can exist independently (e.g., Student and College).
class Student {
int id;
String name;
String school_name;
//create constructor
System.out.println(“\nStudent name is “ + name);
System.out.println(“Student Id is “ + id);
System.out.println(“Student belongs to the “ + school_name + “School”);
}}
class school {
String schoolName;
int noOfStudents;
// create constructor
}
public class AggregationClass
public static void main(String[] args) {
Student n1 = new Student(1, “Shekher”, “Galgotia”);
Student n2 = new Student(2, “Trilok”, “IEC”);
Student n3 = new Student(3, “Aryan”, “ABC”)
}}
- Composition: A strong relationship where one object cannot exist without the other (e.g., Department and College).
Benefits of Association:
- Code reusability
- Cost-effectiveness
- Reducing redundancy
7. What is JDK?
The JDK (Java Development Kit) is a software development kit used to develop Java applications. It includes tools like the Java compiler, Java Runtime Environment (JRE), and APIs needed to create and run Java programs.
JDK (Java Development Kit):
The JDK is a software package used by developers to build Java programs and applications. It includes development tools like the Java compiler (javac
), the Java runtime (java
), and documentation tools (javadoc
), among others.
JRE (Java Runtime Environment):
The JRE is a software package used by clients who run Java applications but don’t develop them. It includes the Java Virtual Machine (JVM) and Java libraries, allowing Java programs to execute on various platforms.
JVM (Java Virtual Machine):
The JVM is responsible for converting Java bytecode (from .class
files) into machine code that can be executed by the host system. It acts as an interpreter between the compiled Java code and the machine’s operating system.
What is JVM and How Does it Work (Architecture)?
The JVM is a crucial component of Java’s platform independence. It handles the execution of Java programs by interpreting compiled bytecode and managing system resources. Key components of the JVM architecture include:
- Class Loader: Loads class files into memory.
- Memory Management (Heap, Stack): Allocates and manages memory for objects and variables.
- Execution Engine: Converts bytecode to machine-specific code and executes it.
- Garbage Collector: Automatically removes unreferenced objects from memory to improve performance.
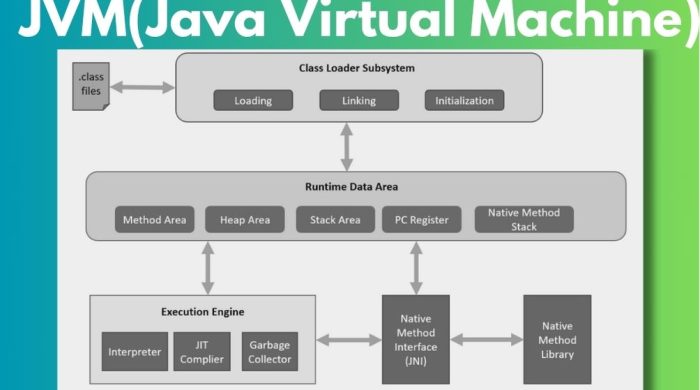
Here’s the optimized version of your content for Google SEO, focusing on user experience, eliminating duplicate keywords, and enhancing readability:
JDK (Java Development Kit)
The JDK is a software package used by developers to build Java programs and applications. It includes development tools like the Java compiler (javac
), the Java runtime (java
), and documentation tools (javadoc
), among others.
JRE (Java Runtime Environment)
The JRE is a software package used by clients who run Java applications but don’t develop them. It includes the Java Virtual Machine (JVM) and Java libraries, allowing Java programs to execute on various platforms.
JVM (Java Virtual Machine)
The JVM is responsible for converting Java bytecode (from .class
files) into machine code that can be executed by the host system. It acts as an interpreter between the compiled Java code and the machine’s operating system.
What is JVM and How Does it Work (Architecture)?
JVM have 3 Parts :
a). Classloader:
Class loader is a subsystem of JVM used to load class data. Whenever we run a Java program, it is first loaded by the class loader. There are 3 classes of functions in Java.
1. Bootstrap ClassLoader: It loades the all core related jar files to run the perticular program or application.
2. Extension ClassLoader: Installs jar files to $JAVA_HOME/jre/lib/ext directory..
3. Application ClassLoader: It,s loads the .class files from classpath.
b). Memory:
1) Class(Method) Area: it contains the class level variables and methods of class.
2) Heap: It contains java objects of a class.
3) Stack: It contains the local variables of a class.
4) Program Counter Register: It is contains the address of current executed instruction of JVM.
5) Native Method : It contains all the native methods, which is used to communicate Java to other languages.
c). Execution Engine:
It contains:
1.A virtual processor
2). Interpreter: it convert .class files into Machine or output language.
3). Just-In-Time(JIT) compiler: It is another compiler, Which is use to increase the speed of Interpreter.
Java Native Interface:
Java Native Interface (JNI) is a framework which is used to communicate with another language like C, C++, .net, Python extra.
8:What are the primitive and Non primitive data members in java?
Primitive: Which is already define by technology, that is known as primitive data members. There are 8 types primitive data member in java.
Example: Byte , short , integer, long , char , Boolean , float, double
Non primitive: –Which is manually created by developer. Exam: Array , String
9: What are the Important Java Keywords ? (Most Imp Question)
I) Static keyword : We have static to save the memory and we don’t need create object and also Static always invoke at class-Loading time so it will print things before main () .
Static keyword used with variable, method and block.
Note: It is share only one time memory.
10: What is static variable? (Most Imp Question)
If a variable is static then it will share all Objects of that class. You just need one time write one time and It will share
Exam: static collage-Name = “IEC engg & Technology, Noida” so this object(collage Name) will share with all students objects.
11: What is static method?
A static method means you don’t need to create a Object to call the static method, it is directly call by class name.
Exam– b Main method of class is a example of static method so don’t need create a object to call this main method, JVM call itself.
public static void main(String[] args) {}
12: What is the static block?
Static block initialize the static data member within a class.
class StaticBlock_Demo {
static { System.out.println(“This is static block”); }
public static void main(String args[]){
System.out.println(“”);
}}
int age;
String name;
Student(int age, String name) {
this.age = age;
this.name = name;
}
void display() {
System.out.println(age + ” “ + name);
}}
class ThisKeyword_Demo {
public static void main(String args[]) {
Student s1 = new Student(25, “ankit”);
Student s2 = new Student(30, “sumit”);
s1.display();
s2.display();
}}
21). Super Keyword: The use of super keyword is, to get the property of Parent class into Child class by forcefully.
Suppose if you get your parent property forcefully then you can get by super keyword in java.
22). Final Keyword: Final keyword use to restrict the user accessibility( means you can’t access that variable for perticular task).
It restrict the access by 3 types in Java:
23). Volatile keyword: If you make a variable volatile, it means it will save in main memory. Benefit is that if 2 or more thread are using same variable form cache memory may some thread modify the value of variable and that variable is accessing by multiple thread, so it will effect to other thread values.
Note: Variable was made as thread safe by using this keyword.Variable was made as thread safe by using this keyword.This keyword also used to make Variable as thread safe.