Design patterns can speed up the development process by providing a way to choose design structures that help developers create scalable, maintainable,and flexible software by providing proven solutions to common problems in a structured way.
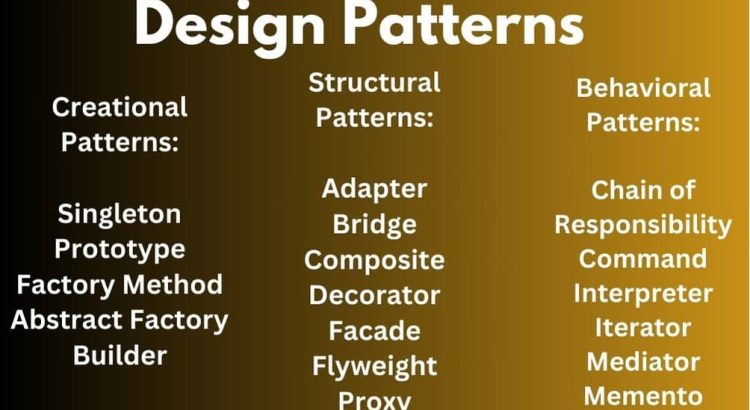
Table of Contents
Toggle1). What is SOLID principles in Java ? or First 5 Principles of Object Oriented Design. (Most Imp Question)
SOLID principles in Java: SOLID principles are an object-oriented approach that are applied to software structure design. It conceptualized by Robert C. Martin (also known as Uncle Bob). These five principles have changed the world of object-oriented programming, and also changed the way of writing software.
SOLID stands for : Single , Open, Liskov, Interface, Dependency
1). SR-P(Single Responsibility Principle ): At a time you need do only task in class , Package or Api. because we need loose coupling.In Every Java class must perform a single functionality. Implementation of multiple functionalities in a single class mashup the code
2). OC-P(Open Close Principle): Application should be open for extension and close for Modification. it means you need to extends class or implements the interface don’t do new modification in calss.
3). LS-P(Liskov Substitution Principle): derived classes must be completely substitutable for their base classes. In other words, child class should be follow the parent class behavior in write manner.
4). IS-P-(Interface Segregation Principle): The larger interfaces split into smaller ones.
5). DI-P(Dependency Inversion Principle): You need depend on Interface and Abstract class instead of Concrete class. It is mandatory for loose coupling.
2). What are Design Patterns? or What are Java Design Patterns?
Design Pattern or Java Design Pattern is a reusable & general(or common) solution to a recurring problem in software design.
or
A design patterns are best-proved solution for solving the specific problem or task.
Like as: Suppose you want to create a class for which only a single instance (or object) should be created and that single object can be used by all other classes. so we will use Sigleton design pattern.
3). What is advantage of Design Patterns ?
These are advantage of Design Patterns:
1. They are reusable in multiple projects.
2. They provide transparency to the design of an application.
3. They provide the solutions that help to define the system architecture.
4. They capture the software engineering experiences.
5. They are well-proved and testified solutions since they have been built upon the knowledge and experience of expert software developers.
6. Design patterns don?t guarantee an absolute solution to a problem. They provide clarity to the system architecture and the possibility of building a better system.
4). What are the different categories of Design Patterns? (Imp Questions)
There are 4 types Design patterns :
1. Creational Patterns : These are discribe, how to object will created at time of class initialize. They can be further classified into object creational and class creational patterns.
Eample: Singleton , Abstract Factory, Factory Method , Prototype, Builder
2. Structural Patterns: This design patterns are concerned with class and object composition in order to create larger structures. They work by identifying and simplifying the relationships between the entities.
Example: Adapter,Bridge,Composite,Decorator,Facade,Flyweight,Proxy
3. Behavioral Patterns: This design patterns are more concerned with identifying the way in which objects communicate with each other.
Example: Chain of Responsibility, Command,Interpreter, Iterator, Mediator, Memento, Observer, State, Strategy, Template Method, Visit
4. J2EE Pattern: It means Java 2 Platform, Enterprise Edition.
Example: MVC (Model-View-Controller)
5). What is Singleton Design Pattern ? (Most Imp Questions)
Singleton design pattern in Java: A class that contains only one instance and provide global access.
or
it restricts the instantiation of a class to a single object and provides a way to access that instance from any point in the application.
6). What is the advantage of singleton design pattern ?
Advantage of Singleton design pattern:
. To save memory because object is not created at each request. Only single instance is reused again and again.
Usage of Singleton design pattern:
. Singleton pattern is mostly used in multi-threaded and database applications. It is used in logging, caching, thread pools, configuration settings et
7). How to create a Sigleton class ? (Most Imp Question)
By these 3 features we can create a sinleton class:
. Static member: It gets memory only once because of static, it contains the instance of the Singleton class.
. Private constructor: It will prevent to instantiate the Singleton class from outside the class.
. Static factory method: This provides the global point of access to the Singleton object and returns the instance to the caller.
Example of a Singleton in Java:
public class Singleton_Example {
// Private static instance
private static Singleton_Example instance;
// Private constructor to prevent external instantiation
private Singleton_Example() {
// Initialization code, if needed
}
// Public method to access the singleton instance
public static Singleton_Example getInstance() {
// Create the instance if it doesn’t exist
if (instance == null) {
instance = new Singleton_Example();
}
return instance;
}}
Singleton pattern object can be created by 2 ways:
Eager Loading: In Eager loading, the object of singleton class gets created when the class is loaded into the memory.
This method of implementation should be avoided as it creates the object even when it is not requested or used.
Lazy Loading: In Lazy loading, the object of singleton class is created when it is actually requested by the client. In this method,
the object is created within the singleton instance accessor method. This is the desired way to create the singleton object as it will
create the instance only when it is required by the client.
8). What is Prototype Design Pattern?
Prototype Design Pattern:
Cloning of an existing object instead of creating new one and can also be customized as per the requirement.
Note: This pattern should be used When the cost of creating a new object is expensive and resource intensive.
Advantage of Prototype Pattern:
In this design pattern you don’t need sub-classes.
o It reduce the complexities of creating objects.
Usage of Prototype Pattern:
o When the classes are instantiated at runtime.
o When the cost of creating an object is expensive or complicated.
o When you want to keep the number of classes in an application minimum.
9). What is Factory Method design Pattern?
Factory Method Pattern: Subclasses are responsible to create the instance of the class.
Advantage of Factory Design Pattern:
. It allows the sub-classes to choose the type of objects to create.
. It provides loose-coupling.
Example: You have create a Interface which is contains shape() and this interface is implement by class circle or triangle or slender and define the shape() body or make abstract method.
10). What is Abstract Factory design pattern ?
Abstract factory design pattern means when a class returns a factory of classes.
Advantage of Abstract Factory Pattern:
. It keep isolates the client code from concrete or implementationclasses.
. It eases the exchanging of object families.
Usage of Abstract Factory Pattern:
. When the system needs to be independent of how its object are created, composed, and represented.
. When the family of related objects has to be used together, then this constraint needs to be enforced.
Eample: Like Factory of Cars create two types cars , Luxury and Ordinary Cars.
11).What is Builder Design Pattern ?
Builder Design Pattern: Builder Pattern says that “construct a complex object from simple objects using step-by-step approach
Advantage of Builder Design Pattern:
. It provides clear separation between the construction and representation of an object.
. It provides better control over construction process.
12). What is Builder Object Pool design Pattern ?
Object Pool Pattern: Object Pool Pattern says that to reuse the object that are expensive to create.
An Object pool is a container which contains a specified amount of objects. When an object is taken from the pool, it is not available in the pool until it is put back. Objects in the pool have a lifecycle: creation, validation and destroy.
13). How many ways can you write singleton class in Java? (Imp Question)
There are 4 ways to create Singleton class in Java:
. Singleton with public static final field initialized during class loading
. Singleton generated by static nested class, also referred as singleton holder pattern
. Singleton by synchronizing get instance () method
. From Java 5 on-wards using Enums
14). Describe the MVC (Model-View-Controller) design pattern.
MVC stands for Model, View and Controller and. It is a software architectural pattern that represt the 3 layer, Model (data and business logic), View (user interface), and Controller (handles user input and updates the Model and View).