Java collections framework | java collections interview questions and answers | java collections interview questions | What is in collection Java?
Collection Datastructure is most hot topic during the Interview. Mostly questions asked from List, Set, Map. Different between List & Set or ArryList & LinkList or Comprable & Comparator. HashMap & Concurrent HashMap also important topic within the collection.
Here are some commonly asked Java Collections interview questions and answers to prepare you for your interview. We are sharing the java collections interview questions, Like as : java collections framework or java collections interview questions and answers or java collections interview questions or What is in collection Java?
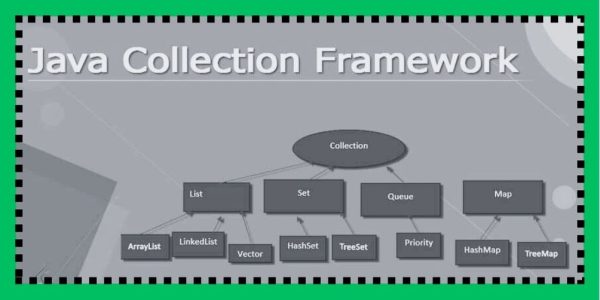
1). What is a Collections Framework? (Imp Question)
Java Collections is the pre defined set of classes and interfaces that help to developer to perform different data operations.
Like as: sorting, searching , traversing, listing extra
Note1: Collections and Array are the data structure of Java.
Note2: The most interface in Collection is Iterable.
2). What are the benefits of the Java Collections Framework?
Benefits of Collections Framework:
I). Improves program quality and speed
II). Increases the chances of reusability of software
III). Decreases programming effort.
IV). Remove the boiler code.
3). What is different between collection and collections ? (Most Imp Question )
Collections: A collection is a class which provides methods to operate on the collection. example — ArrayList, LinkedList, HashMap
Collection: Collection is an interface which represents a group of objects in a single unit. example — List, Set, Queue, Map
Note: Both are present in java.util package and part of the java collections framework.
4). What are the different types of collections in Java? (Imp Question )
There are many different types of collections in Java but some of the most common types of collections include:
Lists: Lists are ordered collections that allow duplicate elements.
Sets: Sets are unordered collections that do not allow duplicate elements. They are often used to store unique values.
Maps: Maps are unordered collections that associate keys with values. They are often used to store data in a key-value format, such as a dictionary or a database.
5).What is the difference between a List and a Set? (Most Imp Question )
List: A list is an ordered collection of elements. It can contain duplicate elements.
Set: A set is an unordered collection of distinct elements. It cannot contain duplicate elements.
5). What is ArrayList in Java? (Most Imp Question )
Java ArrayList class like an array but at the runtime it dynamically increase or decrease the array size & elements.
Array fixed in size.
Note: We can add or remove elements anytime. So, it is much more flexible than the traditional array.
6). What is the difference between an Array and an ArrayList?
Array: An array is a fixed-size data structure that can store elements of the same type. Arrays are declared using the [] syntax. For example, the following code declares an array of integers:
int[] numbers = new int[5];
ArrayList: An ArrayList is a variable-size data structure that can store elements of the same type. ArrayLists are declared using the ArrayList class.
Example:
public class ArrayList_exam {
public static void main(String[] args) {
ArrayList<String> number = new ArrayList<>();
number.add(“Shekher”);
number.add(“Trilok”);
number.add(“Aryan”);
number.add(“Sanjay”);
number.remove(0); // remove 0 index element
number.add(0, “Nannu”);// add at 0 index
// number.clear(); // remove all elements
System.out.println(“Names of Brothers : “ + number);
}}
7). What is LinkedList in java ?
LinkedList also same things like Arraylist but it is maily use to manuplate the elements.
Example:
public class Linkedlst_exam {
public static void main(String[] args) {
LinkedList<String> ls = new LinkedList<>();
ls.add(“Shekher”);
ls.add(“Trilok”);
ls.add(1, “Aryan”);
//forEach loop we store elements one by one and print
for (String string : ls) {
System.out.println(” Names are : “ + string);
}}}
8). What is different between ArrayList & LinkList ? (Imp Question)
These the basic differences between ArrayList & LinkList:
ArrayList |
LinkedList |
1) ArrayList internally work as dynamic |
LinkedList internally work as doubly |
2) Manipulation with ArrayList is slow because |
Manipulation with LinkedList is faster |
3) An ArrayList class can act as a list because |
LinkedList class can act as a list and |
9).What is the difference between a HashMap and a Hashtable? (Imp Question)
|
Hashtable |
A non-synchronized map that allows one |
A synchronized map that does not allow |
It is not thread-safe |
It is thread-safe |
It can’t be shared between multiple threads |
It can be can be shared between multiple threads |
10). Difference between a synchronized collection and an unsynchronized collection in Java:
Synchronized collection:
A collection that is protected by a lock, which ensures that only one thread can access the collection at a time. This prevents race conditions and other concurrency issues. This is also called a thread-safe collection.
Unsynchronized collection: A collection that is not protected by a lock. This means that multiple threads can access the collection at the same time, which can lead to race conditions and other concurrency issues. This is also called a non-thread-safe collection.
11). What is the difference between a concurrent collection and a non-concurrent collection?
Concurrent collections: This type are designed to be accessed by multiple threads simultaneously without causing any data corruption or loss. They do this by using various techniques such as locking, synchronization, and atomic operations.
Non-concurrent collections: This type designed not accessed by multiple threads simultaneously. If multiple threads try to access a non-concurrent collection at the same time, it can cause data corruption or loss.
12). What is the difference between Map and Set? (Imp Question )
Map object has unique keys each containing some value, while Set contains only unique values.
13). What is an iterator?
The Iterator is an interface. It is found in java.util package. It provides methods to iterate over any Collection.
14). What is the difference between Iterator and Enumeration? (Imp Question )
The main difference between Iterator and Enumeration is that Iterator has remove() method while Enumeration doesn’t.
Hence, using Iterator we can manipulate objects by adding and removing the objects from the collections.
Enumeration behaves like a read-only interface as it can only traverse the objects and fetch it.
15). Which methods you need to override to use any object as a key in HashMap?
To use any object as a key in HashMap, it needs to implement equals() and hashCode() method.
16). What is the difference between Queue and Stack?
The Queue is a data structure that is based on FIFO ( first in first out ) property. An example of a Queue in the real-world is buying movie tickets in the multiplex or cinema theaters.
The Stack is a data structure that is based on LIFO (last in first out) property. An example of Stack in the real-world is the insertion or removal of CD from the CD case.
17). What is ConcurrentHashMap and how does it work? (Most Imp Question )
he ConcurrentHashMap allows full concurrency for reads, meaning that any given number of threads can read the same key simultaneously.
It is only getting locked while adding or updating the map. So ConcurrentHashMap allows concurrent threads to read
the value without locking at all. This data structure was introduced to improve performance.
ConcurrentHashMap is a thread-safe implementation of the Map interface in Java,
The read operation can be very fast when the write operation is done with a lock. It provides No object-level Locking.
Example:
public class ConcurrentHashMapDemo {
public static void main(String[] args) {
ConcurrentHashMap<Integer, String> con = new ConcurrentHashMap<>();
// Insert mappings using
con.put(100, “Shekher”);
con.put(101, “Trilok”);
con.put(102, “Aryan”);
con.put(103, “Sanjay”);
// update
con.putIfAbsent(104, “Abhay”);
System.out.println(con);
}}
18). What is the difference between HashSet and TreeSet? (Imp Question )
Main differences between HashSet and TreeSet are :
I). HashSet maintains the inserted elements in random order while TreeSet maintains elements in the sorted order
II). HashSet can store the null object while TreeSet can not store the null object.
19). What is the difference between HashMap and ConcurrentHashMap? (Most Imp Question )
This is also one of the most popular java collections interview questions. Make sure this question is in your to-do list before appearing for the interview.
Main differences between HashMap and ConcurrentHashMap are :
I). HashMap is not synchronized while ConcurrentHashMap is synchronized.
II). HashMap can have one null key and any number of null values while ConcurrentHashMap does not allow null keys and null values.
Feature |
HashMap |
ConcurrentHashMap |
Thread-Safety |
Not |
Thread-safe |
Performance |
Faster for single thread operation but |
Slower |
Data |
Hash |
Hash |
Locking |
Uses |
Uses |
Modification |
Not |
Suitable |
20). How TreeMap works in Java?
TreeMap internally uses a Red-Black tree to sort the elements in a natural order.
21). What is hash-collision in Hashtable? How it was handled in Java? (Most Imp Question )
In Hashtable, if two different keys have the same hash value then it leads to hash -collision. A bucket of type linkedlist used to hold the different keys of same hash value.
22). Explain about the hashCode() and equals() method ? Explain the contract also? (Most Imp Question )
HashMap object uses a Key object hashCode() method and equals() method to find out the index to put the key-value pair. If we want to get value from the HashMap same both methods are used. Somehow, if both methods are not implemented correctly, it will result in two keys producing the same hashCode() and equals() output. The problem will arise that HashMap will treat both outputs the same instead of different and overwrite the most recent key-value pair with the previous key-value pair.
Similarly, all the collection classes that do not allow the duplicate values to use hashCode() and equals() method to find the duplicate elements. So it is very important to implement them correctly.
Contract of hashCode() and equals() methods:
I). If object1.equals(object2) , then object1.hashCode() == object2.hashCode() should always be true.
II). If object1.hashCode() == object2.hashCode() is true does not guarantee object1.equals(object2)
23). What is EnumSet in Java?
EnumSet in=troduced in JDK 1.5. and It is a specialized Set implementation for use with enum types. All of the elements in an enum set must come from a single enum type that is specified explicitly or implicitly when the set is created.
The iterator never throws ConcurrentModificationException and is weakly consistent.
Advantage over EnumSet:
All basic operations of EnumSet execute in constant time. It is most likely to be much faster than HashSet counterparts.
24). What are concurrentCollectionClasses?
It is introduced in jdk 1.5 and it consit into java.util.concurrent Package that has thread-safe collection classes as they allow collections to be modified while iterating. The iterator is fail-safe that is it will not throw ConcurrentModificationException.
Some examples of concurrentCollectionClasses are :
I). CopyOnWriteArrayList
II). ConcurrentHashMap
25). How do you convert a given Collection to SynchronizedCollection?
One line code : Collections.synchronizedCollection(Collection collectionObj) will convert a given collection to synchronized collection.
26). What is IdentityHashMap?
IdentityHashMap is a class present in java.util package. It implements the Map interface with a hash table, using reference equality instead of object equality when comparing keys and values.
In other words, in IdentityHashMap two keys, k1 and k2 are considered equal if only if (k1==k2).
IdentityHashMap is not synchronized.
Iterators returned by the iterator() method are fail-fast, hence, they will throw ConcurrentModificationException.
27). What is WeakHashMap? (Imp Question )
WeakHashMap is a class present in java.util package similar to IdentityHashMap. It is a Hashtable based implementation of Map interface with weak keys. An entry in WeakHashMap will automatically be removed when its key is no longer in ordinary use.
More precisely the presence of a mapping for a given key will not prevent the key from being discarded by the garbage collector.
It permits null keys and null values.
Like most collection classes this class is not synchronized. A synchronized WeakHashMap may be constructed using the Collections.synchronizedMap() method.
Iterators returned by the iterator() method are fail-fast, hence, they will throw ConcurrentModificationException.