Java Exception Handling Interview Questions and Answers (2025)
If you are not handle the Exception in Program that means at the same line program flow will stop.So risky or logic code contains into Try block , handle code contains into Catch block and if exception not handle than we use Finally block.
Here, we have listed the most important exception handling interview questions in Java with the best possible answers.These interviewer can ask you different questions, Like as: java exception handling or what is java exception handling or Java Exception Handling Interview Questions or types of exception handling in java, Java Exception Handling | what is java exception handling | Java Exception Handling Interview Questions | types of exception handling in java extra…
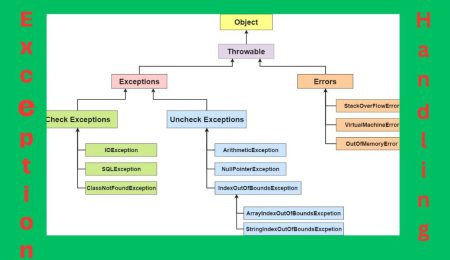
1) What is an Exception in Java? Most Imp Question for fresher
An exception in Java is an unexpected or abnormal situation that occurs while the program is running. It can cause the program to stop if not handled properly.
Example:
public class ExceptionDemo {
public static void main(String[] args) {
try {
int a = 10 / 0; // Causes an ArithmeticException
} catch (Exception e) {
System.out.println("Exception handled by catch block");
}
System.out.println("The main method is running, and the program continues.");
}
}
In the example, dividing by zero throws an exception, but it’s caught and handled to prevent the program from stopping abruptly.
2) What is the Superclass of All Exceptions in Java?
The superclass of all exceptions in Java is the Exception
class.
3) What is the Top-Level Class for Errors and Exceptions in Java? (Most Important Question)
Throwable
is the top-level class for both errors and exceptions in Java.
4) What is Exception Handling in Java? (Important Question)
Exception handling in Java ensures that the program continues running smoothly even if unexpected situations (errors) occur. It is done using try
, catch
, and finally
blocks to handle exceptions like ClassNotFoundException
, FileNotFoundException
, IOException
, etc.
5) What is the Difference Between Error and Exception in Java? (Most Important Question)
- Errors: Caused by the Java Virtual Machine (JVM) environment. Example:
OutOfMemoryError
. - Exceptions: Caused by the application itself. Example:
NullPointerException
.
Errors only occur at runtime, while exceptions can occur at both compile time and runtime.
6) How are Exceptions Handled in Java? (Important Question)
Java handles exceptions using three blocks:
- try: Contains the risky code that might throw an exception.
- catch: Catches the exception thrown by the
try
block. - finally: Always executes, regardless of whether an exception was thrown or not.
7) Does the Finally Block Always Get Executed in Java? (Important Question)
No, the finally
block won’t execute if the program is terminated using System.exit(0)
.
8) What is the Advantage of Using Exception Handling in Java?
- Ensures smooth execution of the program.
- Allows customization of error messages.
- Separates regular code from error-handling code, making programs cleaner.
9) What is the Difference Between Checked and Unchecked Exceptions in Java? (Most Important Question)
- Checked Exceptions: These exceptions are checked at compile-time. Example:
IOException
,SQLException
. - Unchecked Exceptions: These exceptions are not checked at compile-time, but they are checked at runtime.
- Example:
ArithmeticException
,NullPointerException
.
10) What is the Difference Between final
, finally
, and finalize
in Java? (Most Important Question)
- final:
- For variables: Cannot be reassigned.
- For methods: Cannot be overridden.
- For classes: Cannot be extended.
- finally: A block of code that always runs after the
try
andcatch
blocks. - finalize: A method called by the garbage collector before destroying an object.
11) Can We Throw an Exception Manually in Java? (Important Question)
Yes, you can throw an exception manually using the throw
keyword.
Syntax:
throw new ExceptionType("Message");
12) What Are the Different Types of Exceptions in Java? Important Question for fresher & Exp.
- ArithmeticException: Occurs when an illegal arithmetic operation happens (e.g., division by zero).
- NullPointerException: Occurs when trying to use an object that is
null
. - NumberFormatException: Occurs when attempting to convert a string to a number, but the string isn’t a valid number.
Example:
String s = "abc";
int i = Integer.parseInt(s); // Throws NumberFormatException
13) What Are the Keywords in Java for Exception Handling? Imp Question for fresher
The main keywords for handling exceptions in Java are:
throw
,throws
,try
,catch
, andfinally
.
14) What is a Custom Exception in Java? (Most Important Question)
A custom exception is a user-defined exception that allows you to define your own error messages for specific conditions in your application.
Example:
class MyException extends Exception {
public MyException(String message) {
super(message);
}
}
public class CustomExceptionExample {
public static void main(String[] args) {
try {
throw new MyException("Business logic failed during account verification");
} catch (MyException ex) {
System.out.println("Caught: " + ex.getMessage());
}
}
}
In this example, a custom exception (My=xception
) is created and thrown with a specific message.
15) What is the Difference Between throws
and throw
in Java?
Most Imp question for fresher & experience .
-
throw
: Used to explicitly throw an exception from a method or block of code. Example:throw new ArithmeticException("Cannot divide by zero");
-
throws
: Used in a method signature to declare that a method might throw exceptions. It is followed by a list of exceptions that the method may throw. Example:public void readFile() throws IOException { // Code that might throw an IOException }
16) What is the Use of try-with-resources
in Java? Important Question for fresher
The try-with-resources
statement is used to automatically close resources like files, streams, or database connections after use. This avoids resource leaks by ensuring that resources are closed even if an exception is thrown.
Example:
try (FileReader fr = new FileReader("file.txt")) {
// Code that uses the file
} catch (IOException e) {
System.out.println("Error reading file: " + e.getMessage());
}
// No need to explicitly close FileReader; it is closed automatically
17) What is an Assertion in Java?
Assertions are a debugging aid that helps to test assumptions made by the programmer during development. They are used to verify that a particular condition holds true during runtime.
Example:
int age = -1;
assert age >= 0 : "Age cannot be negative"; // AssertionError is thrown if age is negative
To enable assertions, you need to run the program with the -ea
option (enable assertions).
18) What is a Stack Trace in Java?
A stack trace provides detailed information about the sequence of method calls that led to an exception. It helps developers track down where the exception occurred and the flow of execution that led to the error.
Example:
try {
int result = 10 / 0; // Will throw ArithmeticException
} catch (ArithmeticException e) {
e.printStackTrace(); // Prints the stack trace to help diagnose the error
}
19) Can We Handle Multiple Exceptions in One catch
Block?
Yes, from Java 7 onwards, you can handle multiple exceptions in a single catch
block using the |
(OR) operator.
Example:
try {
// Code that might throw multiple exceptions
} catch (ArithmeticException | NullPointerException e) {
System.out.println("Handled Exception: " + e.getMessage());
}
18) What is the Purpose of the getCause()
Method in Java?
The getCause()
method returns the underlying cause of the exception, which might be another exception that caused the current one. This is useful for chained exceptions.
Example:
try {
// Code that throws a new exception
} catch (Exception e) {
Throwable cause = e.getCause();
if (cause != null) {
System.out.println("Root Cause: " + cause);
}
}
19) What Happens If an Exception is Not Handled? Imp question for fresher
If an exception is not caught or handled, the Java runtime will terminate the program. If the exception is unchecked (like a NullPointerException
or ArrayIndexOutOfBoundsException
), it can lead to abnormal termination of the program.
20) What are the Different Types of Errors in Java?
- Virtual Machine Errors: These errors occur due to issues with the Java Virtual Machine (JVM). Example:
OutOfMemoryError
,StackOverflowError
. - Linkage Errors: These occur when there is a class definition mismatch. Example:
NoClassDefFoundError
,ClassNotFoundException
. - IOErrors: These errors occur due to issues with input/output operations. Example:
IOException
.
21) What is the NoClassDefFoundError
in Java?
NoClassDefFoundError
occurs when a class that was available at compile time is not found at runtime. It usually happens when a class is not on the classpath or there is a version mismatch between compile-time and runtime.
10) What is a ClassCastException
in Java?
ClassCastException
is thrown when you attempt to cast an object to a class of which it is not an instance.
Example:
Object obj = "Hello";
Integer num = (Integer) obj; // This will throw ClassCastException
These questions cover a wide range of Java exception handling topics and are essential for mastering exception management in Java. By understanding these concepts, you’ll be well-equipped to handle errors and exceptions effectively in your Java applications.
By understanding these key concepts of exception handling in Java, you can ensure your programs handle unexpected situations effectively and maintain smooth execution.