Java Multithreading: Essential Concepts and Interview Questions :
Multithreading is a crucial concept in programming, especially in Java, where it plays a key role in improving performance. It involves concurrency and the use of multiple threads to enhance the efficiency of applications. For Java developers, mastering Java multithreading is vital for better resource utilization and optimal program performance.
Understanding Multithreading in Java: In Java multithreading, managing multiple threads simultaneously allows tasks to run concurrently, which significantly boosts an application’s responsiveness and efficiency. Effective multithreading in Java can lead to faster processing times and overall improved performance.
As Java developers, it’s important to dive deep into the topic of multithreading to leverage its full potential. Whether you’re preparing for an interview or refining your coding skills, here are some essential Java multithreading interview questions and answers to help you succeed. Topics include multithreading in Java, Java thread synchronization, and practical Java multithreading examples.
Stay updated with the latest trends and expert insights into Java multithreading interview questions , and ensure you are fully prepared for your next opportunity.
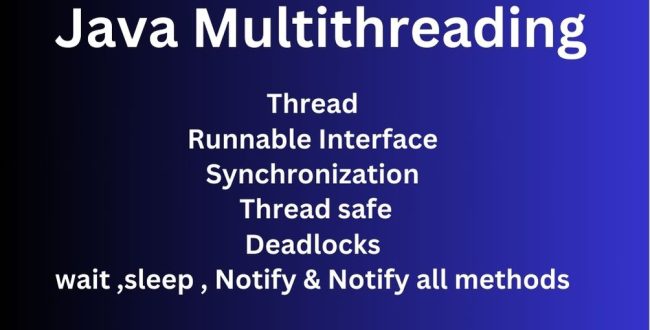
1) What is the Difference Between Multitasking and Multithreading? Imp questions for fresher
Multitasking involves executing multiple tasks simultaneously on a computer, often by switching between tasks rapidly. For example, while you are typing code, downloading software, and listening to music at the same time, you are multitasking.
Multithreading in Java, on the other hand, is the execution of multiple threads concurrently within a single process, enhancing CPU utilization. Each thread shares memory space, allowing faster context switching compared to separate processes.
2) Advantages of Multithreading
- Time Efficiency: Multithreading enables simultaneous execution of multiple tasks, saving time.
- Thread Independence: If one thread encounters an error, it doesn’t affect others, allowing continuous operations without blocking.
3) What is a Thread?
In Java, a thread is a lightweight process that runs in parallel with other threads. By leveraging multiple CPUs, multithreading boosts the performance of Java applications. Threads share memory and resources, enabling improved task execution and better parallelism.
4) Thread vs. Process: What’s the Difference?
A process is a complete execution environment, while a thread is a smaller unit of execution within a process. Threads share the same memory space, enabling efficient communication. In contrast, processes have separate memory spaces, making them more isolated.
5) Life Cycle of a Thread Most imp question for fresher & experience
A thread undergoes five states in its life cycle:
- New: The thread is created but not yet started.
- Runnable: The thread is ready to execute.
- Running: The thread is actively executing.
- Blocked: The thread is waiting for resources.
- Terminated: The thread has completed its task.
6) How to Achieve Multithreading in Java ? Imp question for fresher
There are two ways to create threads in Java:
- Extending the Thread Class:
public class MyThread extends Thread { public void run() { System.out.println("Thread running."); } public static void main(String[] args) { MyThread t = new MyThread(); t.start(); } }
- Implementing the Runnable Interface:
public class MyRunnable implements Runnable { public void run() { System.out.println("Thread running via Runnable interface."); } public static void main(String[] args) { MyRunnable myRunnable = new MyRunnable(); Thread t = new Thread(myRunnable); t.start(); } }
7) Can a Thread Be Started Twice?
No, once a thread is started, it cannot be restarted. Attempting to start a thread again will throw an IllegalThreadStateException
.
8) What is Synchronization in Multithreading?
Synchronization ensures that only one thread can access a resource at a time. This prevents errors caused by simultaneous access to shared resources, known as race conditions. The synchronized
keyword is used to ensure thread-safe execution.
Example:
public synchronized void printTable(int n) {
for (int i = 1; i <= 5; i++) {
System.out.println(n * i);
}
}
9) Benefits of Synchronization
- Thread Safety: Synchronization ensures that shared resources are accessed safely.
- Orderly Execution: Prevents race conditions, ensuring predictable results.
- Deadlock Prevention: Ensures that threads don’t get blocked indefinitely.
10) What is a Race Condition?
A race condition occurs when multiple threads try to access and modify shared data simultaneously, leading to unpredictable results. For example, if one thread is reading data and another thread is deleting the same data, it can cause an inconsistency.
11) How to Achieve Thread Safety?
Thread safety can be achieved using:
- Synchronization
- Volatile keyword
- Immutable objects
- Atomic operations
- Concurrent collections like
Concurrent-HashMap
12) What is Deadlock in Java?
A deadlock occurs when two threads wait for each other to release resources, causing them to get stuck indefinitely. Deadlock prevention requires careful management of locks and resources.
13) What Does Thread-Safe Mean?
A class is thread-safe if it ensures that its methods or variables can be safely accessed and modified by multiple threads simultaneously. This is typically achieved through synchronization or using thread-safe data structures.
14) Difference Between start() and run() in Thread Execution (Imp question for fresher)
start()
: Starts a new thread and calls therun()
method internally.run()
: Executes the code in the same thread, not creating a new one.
15) Best Approach for Multithreading: Runnable Interface or Thread Class? (Imp question for fresher)
It is recommended to implement the Runnable interface rather than extending the Thread class. This approach allows more flexibility (you can extend other classes) and adheres to best practices for multithreading in Java.
16) Callable vs. Runnable: Key Differences
- Callable: Can return a result and throw checked exceptions, making it more powerful.
- Runnable: Simpler, with no return value and no exception handling.
Conclusion: Understanding Java multithreading is essential for optimizing performance and efficiency in your applications. By mastering key concepts like thread synchronization, thread safety, and the difference between threads and processes, you can develop more robust, scalable Java applications. Use the best practices for creating threads, such as implementing the Runnable interface and avoiding thread-related pitfalls like deadlocks.
This version of the content is SEO-optimized, focused on Java multithreading, and ensures readability with human-friendly language while integrating trending keywords like “Java multithreading,” “thread safety,” “race conditions,” and “multithreading interview questions.”