Serialization contains numbers of topics but today era mustly useful & asked by interviewer that is Serialization and Deserialization topic. Serialization to change java objects into Stream objects(or non readable form) and also again convert from Stream objects to java objects by Deserialization.
Serialization holds significant importance in Java interviews, demanding a thorough understanding from Java developers.
It’s crucial for them to be well-versed in responding to serialization-related interview questions. In this article,we will discuss some of the most asked Serialization interview questions, like as : what is serialization in java or what is serialization in java with example or what is serialization in java and why it is used or serialization interview questions.
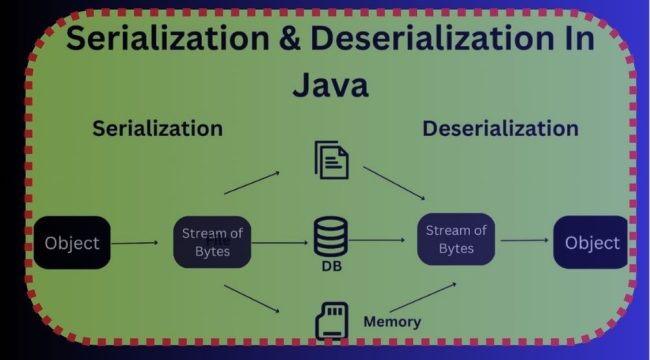
1). What is Serialization in java? (Imp Question)
Serialization is a process in Java which is convert java Objects into stream that can be transferred over the network or save it as file or store in DB for later usage.
2). What is De-serialization in java?
When a stream of bytes transferred over the network so another side of the network needs to revert back to java object. This process is called deserialization.
Example : Below is the Program of Serialization & De-Serialization
class Car implements Serializable {
String name = “Tesla”;
String owner = “Elon Mask”;
int year = 1958;//if u use transient keyword this variable so this variable will not serialized
}
public class SerailIzation_Desericalization_Exam extends Car {
public static void main(String[] args) {
// to make the Serialized
try {
Car myCar = new Car();
String filename=“serializable.ser”;
FileOutputStream fos = new FileOutputStream(filename);
ObjectOutputStream oos = new ObjectOutputStream(fos);
oos.writeObject(myCar);
oos.flush();
oos.close();
System.out.println(“Serialization success”);
} catch (Exception e) {
System.out.println(e);
}
// To make De-Serialized
try {
FileInputStream fis = new FileInputStream(“serializable.ser”);
ObjectInputStream ois = new ObjectInputStream(fis);
Car readCar = (Car) ois.readObject();
System.out.println(“\nDeserialez data below “);
System.out.println(“Car name:” + readCar.name);
System.out.println(“Car owner:” + readCar.owner);
System.out.println(“Car production year:” + readCar.year);
ois.close();
} catch (Exception e) {
System.out.println(e);
}}}
3). Why do we need to use Serialization in java?
Serialization actually needed for transferring the byte stream over the network.
Some real-time applications using java streams are listed below:
I) Data transmission
II) Persistence
III) Deep cloning
IV) Cross JVM communication
V) Stashing
4) How to make a Serialized class in java ? or How can we implement Serialization in java?
By implementing the Serializable interface and implement the Externalizable interface.
5). What is the Marker interface? (Most Imp Question)
A Marker interface is one that has no methods or fields.The Serializable interface is a Marker interface.
JVM automatically identifies whether the class is Serializable by checking the Serializable interface is implemented or not.
Note:Java.io.Serializable and java.lang.Cloneable are some of the examples of marker interface.
6). Why the Serializable interface is called the Marker interface in Java?
The serializable interface has no methods or fields. We know that the interface which does not contain any method is called a Marker interface. That’s why the Serializable interface is Marker Interface.
7). What is serialVersionUID?
When we make a class Serializable we have to declare a final static long variable with a value named by serialVersionUID. This variable will be serialized and passed during the byte stream transfer. During deserialization, the compiler will compare this serialVersionUID with the saved one.
8). How can we restrict some variables to be serialized?
If we want to restrict a variable from serialized, we should declare this variable as a transient variable.