Java Wrapper classes is used to convert primitive data types into objects and it is provide a way to use primitive data types ( int , boolean , etc..) as objects.In Object Oriented Programming Language everything is an object .So in collection topic it requires Wrapper classes convert primitives to objects and primitives to object So for conversion Wrapper classes are used in java
In this post, we will discuss some of the most asked interview questions on wrapper classes. Like as: what are wrapper classes in java or What are wrapper classes in Java interview questions or What is the size of wrapper class in Java?
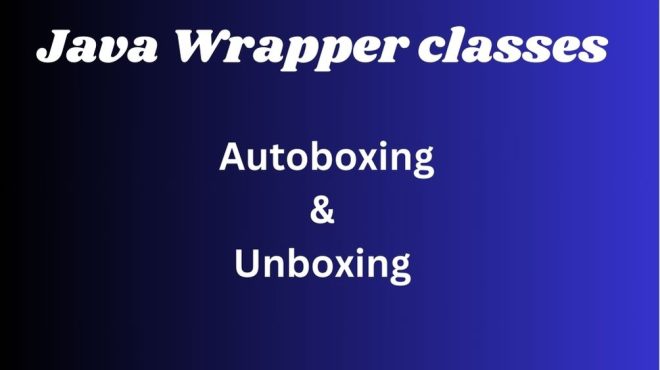
1). What are the Wrapper classes ? (Most Imp Question)
Those classes whose object, contains or wraps primitive data types.
or
A Wrapper Class is any class which wraps or contains the functionality of another class or component. A Wrapper Class that wraps or encapsulates the primitive data type is called Primitive Wrapper Class.
2). What are the Uses of Wrapper classes?
The Uses of Wrapper classess are:
. Wrapper classes helps in serialization. We can convert primitives to Objects using wrapper class.
. Mainly in Collection Framework we are working with objects. Like Array List, Linked List,etc are working with wrapper class only.What are the Uses of Wrapper classes?
3). What is Autoboxing ? (Most Imp Question)
When we convert primitive data into objects that is Autoboxing.
Example:
//Autoboxing example of int to Integer
public class Autoboxing {
public static void main(String[] args) {
int a = 20;
// converting int into Integer
Integer i = Integer.valueOf(a);
Integer j = a;
System.out.println(“From int to Integer or Object value is : “ + j);
}}
4). What is unboxing? (Most Imp Question)
Converting an object into its corresponding primitive datatype is called unboxing.
Example:
public class Unboxing {
//Autoboxing example of int to Integer
public static void main(String[] args) {
//Converting int into Integer
Integer a= new Integer(30);
int i=a.intValue();
int j=a;
System.out.println(“Form Object to Primitive : “+j);
}}
5). What are the Wrapper classes available for primitive types ?
Ans. boolean – java.lang.Boolean
byte – java.lang.Byte
char – java.lang.Character
double – java.lang.Double
float – java.lang.Float
int – java.lang.Integer
long – java.lang.Long
short – java.lang.Short
void – java.lang.Void
6). Difference between boolean and Boolean ?
boolean is a primitive type whereas Boolean is a class.
7). What Design pattern Wrapper Classes implement ?
8). Do All The Wrapper Classes Support Caching?
Yes, all the wrapper classes support caching to increase performance of Autoboxing and Auto-unboxing. But unlike Integer, they have fixed caching size upto 127 only. You can not enhance the range.
People also ask- what java wrapper class | what is the use of java wrapper classes | what are wrapper classes in java explain with example | what are wrapper classes in java and why are they used | what are wrapper classes in java used for | what is wrapper classes in java programming | why do we need java wrapper classes | how many wrapper classes are there in java | how wrapper classes work in java | java wrapper classes vs primitive data types | java wrapper classes and autoboxing | java wrapper class vs string | java wrapper class vs boxing | java wrapper class vs | why do we need java wrapper classes